Authentication in Flutter
Authentication and integrating a database are the two building blocks of any app. We use authentication to make sure users have access only to their own data.
Initial set up
Setting up Firebase Auth as per the docs:
https://pub.dartlang.org/packages/firebase_auth
They also tell us to install Google Sign in:
https://pub.dartlang.org/packages/google_sign_in
Enable Google Sign In on Firebase Auth
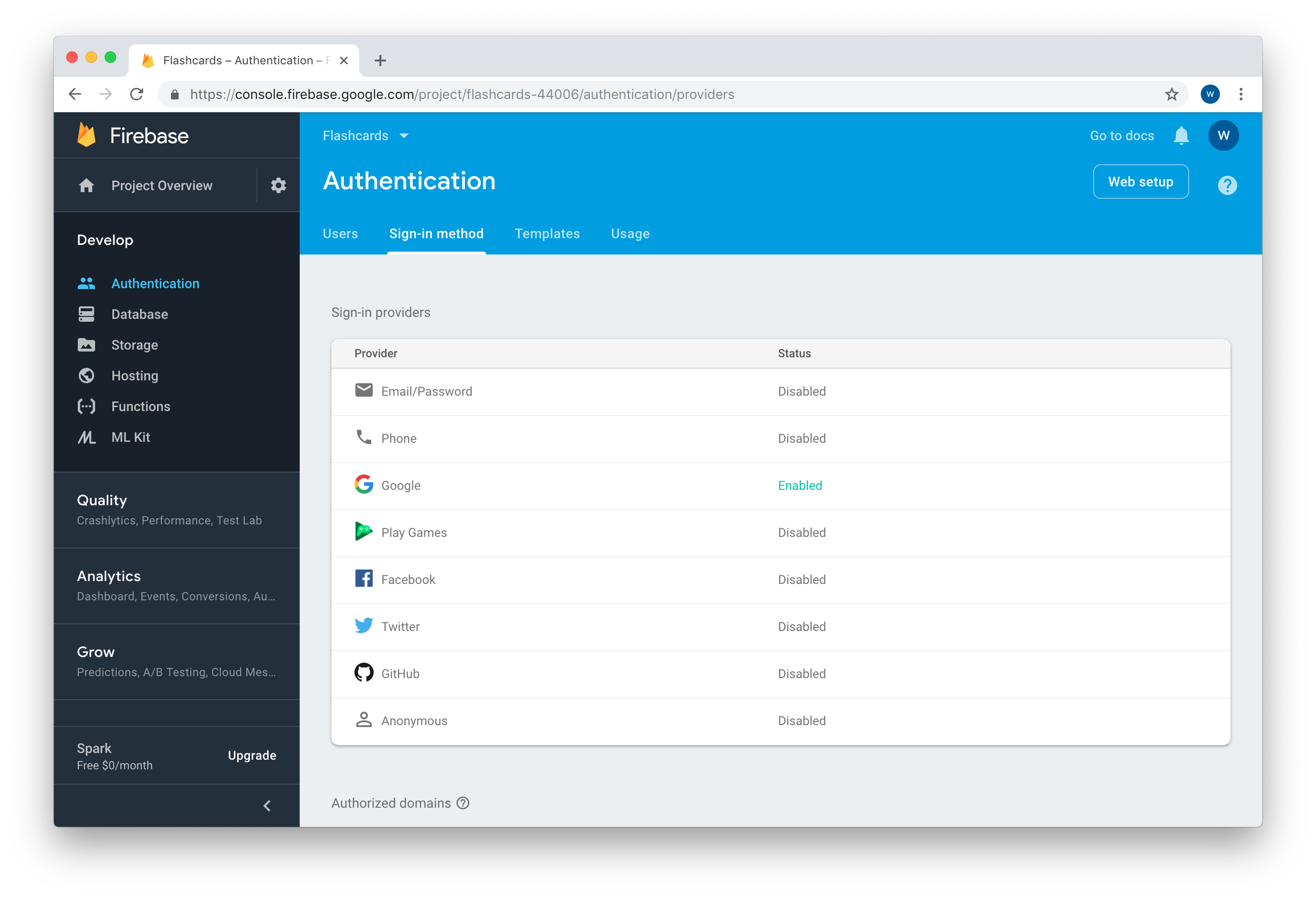
Back to the code
I copy/pasted their _handleSignIn() routine, then added an icon to my appBar. When I tap on the icon, I get the following error in logcat:
W/GooglePlayServicesUtil(14258): Google Play services out of date. Requires 12451000 but found 11947470
According to this post, it means the virtual device has an old version of Google Play Services. I should use a newer version.
In Android Studio's SDK Manager, I had to check "Show package details" and select the update to Google APIs System Image.
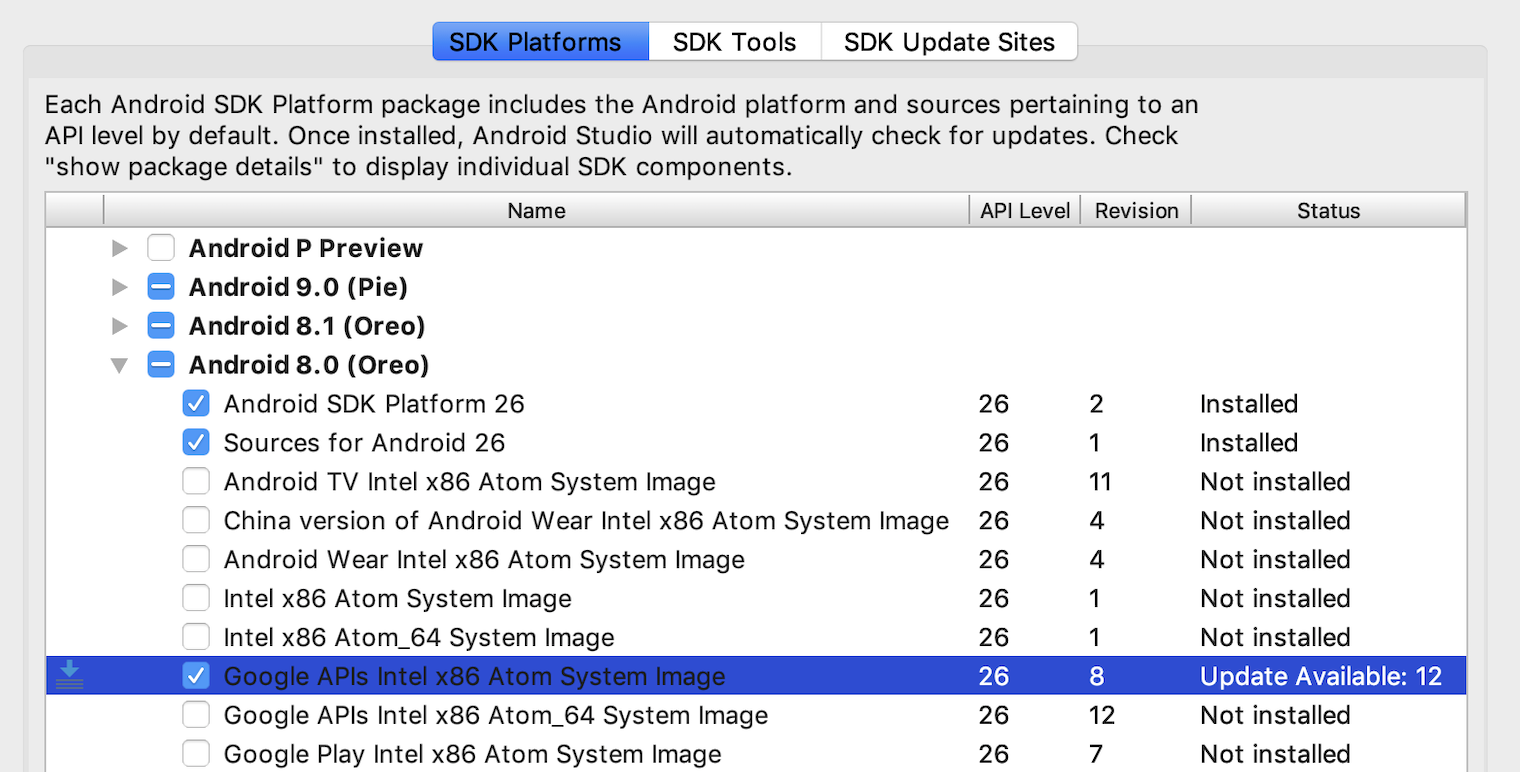
Finally I did a cold boot of the VM. When I tap the icon again, this time it asks for my credentials!
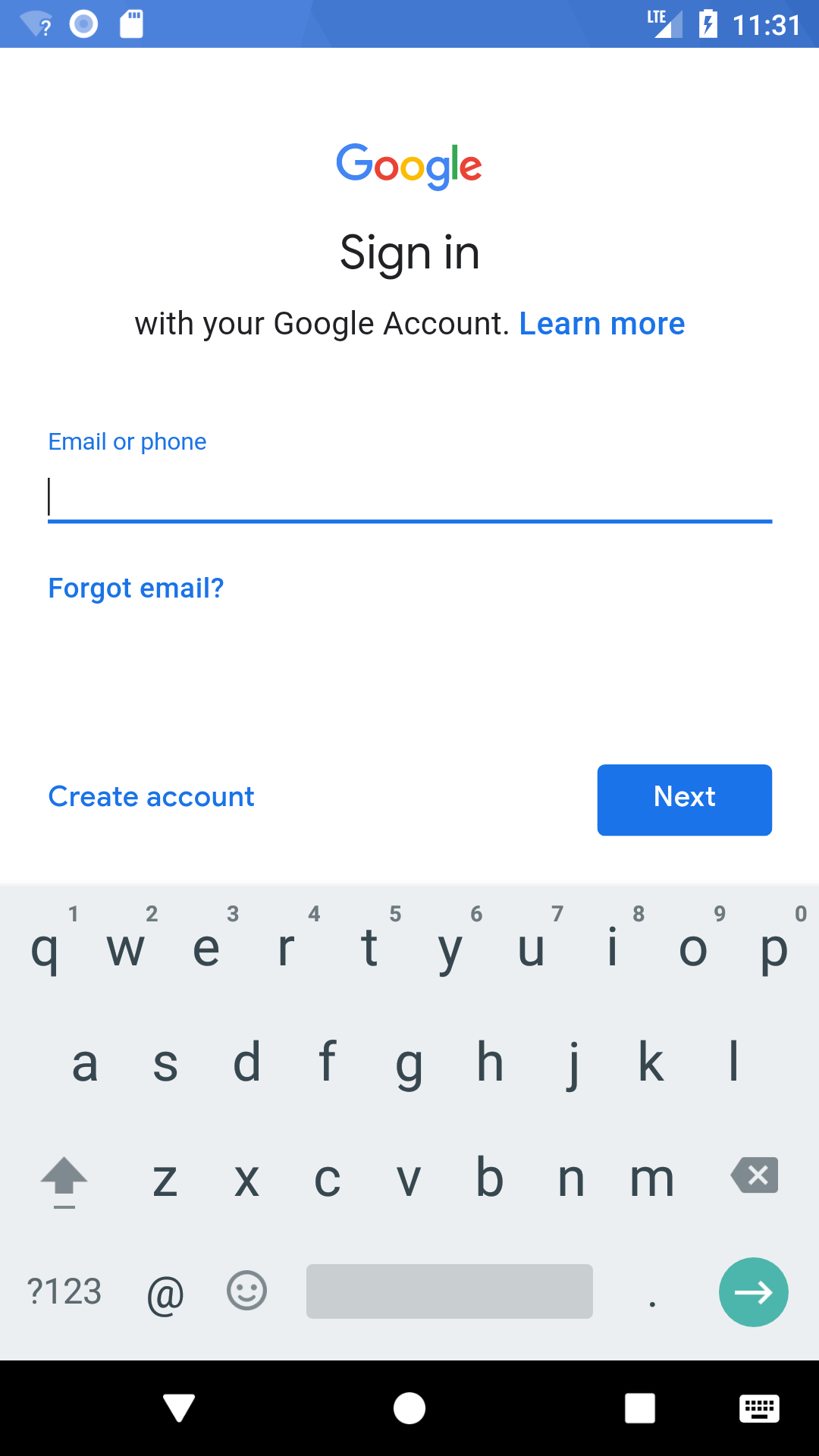
On sign in, it prints my info:
I/flutter ( 4974): signed in [myname]
Showing signed in state
Users only need to sign in once. The sign in data is then cached. So on top of making a sign in button, we should have a listener to change the view whenever sign in state changes. Here's how to do it:
final FirebaseAuth _auth = FirebaseAuth.instance;
...
_auth.onAuthStateChanged.listen((user) {
// If user == null, the user is signed out.
// Otherwise, the user is signed in.
// If the user is signed in, check `user.isAnonymous` for anonymous logins.
});
Protecting user data
We want the user to be able to access only their data. More to come.