Make Prettier work nicely with ESLint in VSC
These days I am writing Firebase Functions, and I encountered this issue where VSC will fix formatting for me, but when I try to deploy the function, ESLint complains.
I had this import in my code:
import { IncomingMessage } from "node:http";
VSC is set up so that Prettier will format on Save, so it will always write the import like above.
Then when I run npm run deploy
, one of the first steps of deployment is to run eslint
. And this fails with the following error:
/Users/anhtuan/git/cargo/functions/src/index.ts
2:9 error There should be no space after '{' object-curly-spacing
2:25 error There should be no space before '}' object-curly-spacing
43:10 error There should be no space after '{' object-curly-spacing
43:22 error There should be no space before '}' object-curly-spacing
✖ 4 problems (4 errors, 0 warnings)
4 errors and 0 warnings potentially fixable with the `--fix` option.
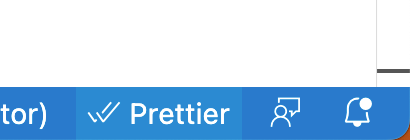
In the lower right corner of VSC, if I click Prettier, I get the following information:
No local configuration (i.e. .prettierrc or .editorconfig) detected, falling back to VS Code configuration
Prettier Options:
{
"arrowParens": "always",
"bracketSpacing": true,
"endOfLine": "lf",
"htmlWhitespaceSensitivity": "css",
"insertPragma": false,
"singleAttributePerLine": false,
"bracketSameLine": false,
"jsxBracketSameLine": false,
"jsxSingleQuote": false,
"printWidth": 80,
"proseWrap": "preserve",
"quoteProps": "as-needed",
"requirePragma": false,
"semi": true,
"singleQuote": false,
"tabWidth": 2,
"trailingComma": "es5",
"useTabs": false,
"vueIndentScriptAndStyle": false,
"filepath": "/Users/anhtuan/git/cargo/functions/src/index.ts",
"parser": "typescript"
}
So what I did is create a file called .prettierrc
in the functions
subfolder, and add this:
{
"bracketSpacing": false
}
Then I saved the file to force VSC to run Prettier again, and it fixed my import to this:
import {IncomingMessage} from "node:http";
Finally I was able to deploy my function.