Using Firebase in Flutter on macOS
Firebase Auth
Authentication methods
I typically use Google Sign In for my projects, but as you can see on https://pub.dev/packages/google_sign_in, it only supports Android, iOS and Web, and there is no GitHub issue tracking Desktop. Phone authentication also required extra steps, and it didn't mention macOS, so I skipped it.
Note: I later found out it might be possible as the instructions for iOS might apply to macOS as well.
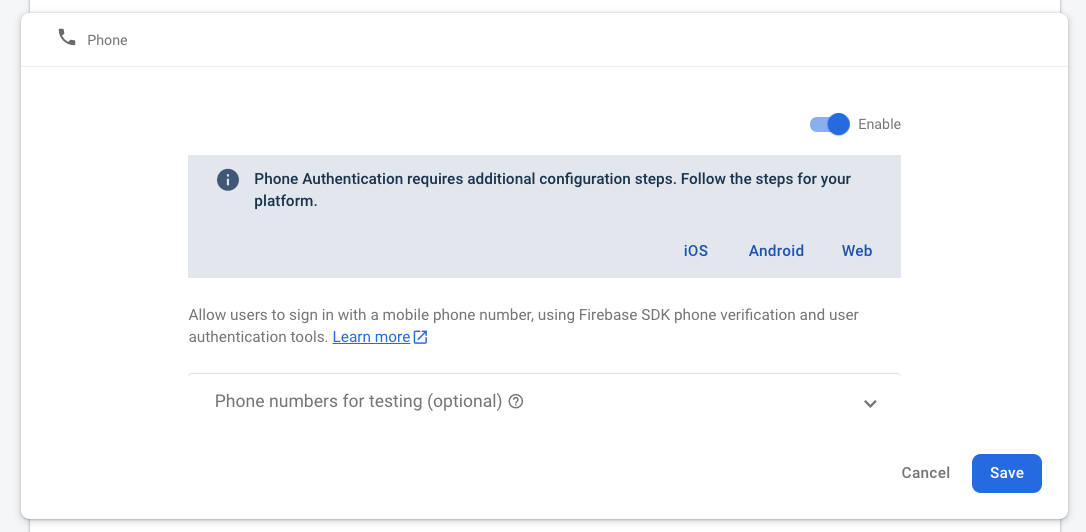
Email and password
After adding the dependency to firebase_core and firebase_auth to my project, and adding a piece of code to sign in, I run my app and get this:
/usr/local/bin/pod:23:in `<main>'
- Update the `platform :osx, '10.11'` line in your macOS/Podfile to version `10.12` and ensure you commit this file.
- Open your `macos/Runner.xcodeproj` Xcode project and under the 'Runner' target General tab set your Deployment Target to 10.12 or later.
Error output from CocoaPods:
↳
[!] The FlutterFire plugin firebase_auth for macOS requires a macOS deployment target of 10.12 or later.
Changed the version in Podfile.
Next, since FirebaseCore is not null-safe, it refused to run again:
[project]/macos/Pods/FirebaseCore/FirebaseCore/Sources/FIRLogger.m:58:28: warning: this old-style function definition is not preceded by a prototype [-Wstrict-prototypes]
void FIRLoggerInitializeASL() {
^
[project]/macos/Pods/FirebaseCore/FirebaseCore/Sources/FIRLogger.m:101:20: warning: this old-style function definition is not preceded by a prototype [-Wstrict-prototypes]
void FIRResetLogger() {
^
2 warnings generated.
Error: Cannot run with sound null safety, because the following dependencies
don't support null safety:
Gotta run my app with unsound null safety. Following the guide on unsound null safety, I add to main.dart:
// @dart=2.9

Upon sign up, I get:
[core/no-app] No Firebase App '[DEFAULT]' has been created - call Firebase.initializeApp()
So I create a "web app" in my project, just so it shows me the settings for initializeApp. I copy paste these settings into my app and run it again. It fires another error.
It turns out I missed and should have read this first: https://firebase.flutter.dev/docs/overview/#iosmacos
Ok let's add the flag to the top of my Podfile. This time it won't launch:
-> Fetching podspec for `FlutterMacOS` from `Flutter/ephemeral`
-> Fetching podspec for `firebase_auth` from `Flutter/ephemeral/.symlinks/plugins/firebase_auth/macos`
firebase_auth: Using user specified Firebase SDK version '8.0.0'
-> Fetching podspec for `firebase_core` from `Flutter/ephemeral/.symlinks/plugins/firebase_core/macos`
firebase_core: Using user specified Firebase SDK version '8.0.0'
Resolving dependencies of `Podfile`
...
* out-of-date source repos which you can update with `pod repo update` or with `pod install --repo-update`.
* changed the constraints of dependency `Firebase/CoreOnly` inside your development pod `firebase_auth`.
You should run `pod update Firebase/CoreOnly` to apply changes you've made.
...
Error: CocoaPods's specs repository is too out-of-date to satisfy dependencies.
To update the CocoaPods specs, run:
pod repo update
Exception: Error running pod install
I ran it and it failed because I have an M1 processor and should use it with arch
:
% arch -x86_64 pod repo update
Updating spec repo `trunk`
It didn't do anything though. Turns out, the actual command is to update Firebase/CoreOnly. The logs above mentioned it, but it was so long I missed it the first time. This time it installed new dependencies fine:
% arch -x86_64 pod update Firebase/CoreOnly
Updating local specs repositories
Analyzing dependencies
firebase_auth: Using user specified Firebase SDK version '8.0.0'
firebase_core: Using user specified Firebase SDK version '8.0.0'
Downloading dependencies
Installing Firebase (8.0.0)
Installing FirebaseAuth (8.0.0)
Installing FirebaseCore (8.0.0)
Installing FirebaseCoreDiagnostics (8.0.0)
Installing FlutterMacOS (1.0.0)
Installing GTMSessionFetcher (1.5.0)
Installing GoogleDataTransport (9.0.0)
Installing GoogleUtilities (7.4.1)
Installing PromisesObjC (1.2.12)
Installing firebase_auth (0.20.1)
Installing firebase_core (0.7.0)
Installing nanopb (2.30908.0)
Generating Pods project
Integrating client project
Pod installation complete! There are 3 dependencies from the Podfile and 12 total pods installed.
When I run it, I get the same error as before:
Firebase has not been initialized. Please check the documentation for your platform.
Then I realize it's because I should probably follow the iOS set up guide, which involves downloading a .plist file. See https://firebase.google.com/docs/ios/setup.
After creating the iOS app in the Firebase Console, downloading the file and adding it to my Runner project, it runs!
When I attempt to sign up, however, I get this:
Network error (such as timeout, interrupted connection or unreachable host) has occurred.
This is probably a permission issue in my MacOS app. A quick search gets me this post where they kindly refer to the official doc: https://flutter.dev/desktop#entitlements-and-the-app-sandbox.
Here's a screenshot of the Signing and Capabilities pane:
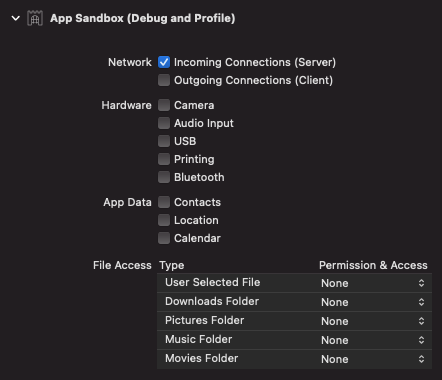
And this time it seems it signs in fine!!
Upon restart, I get this dialog. The app tries to access the keychain:
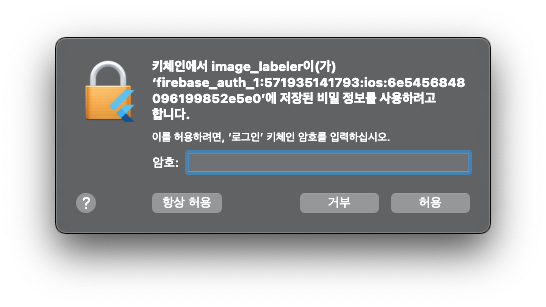
This is to retrieve authentication information. If you've previously signed in, you won't need to sign in again.