Using records and other Dart 3 features in Flutter
Dart 3 alpha recently got announced here. It introduces new features like records (tuples) which I wanted to use. The article also mentions the latest version of Flutter stable ships with Dart 3 alpha.
Enabling experimental features in VSC
To use it, we should change the pubspec.yaml to use SDK >= 3.0.0. I got the Dart version by running flutter --version
:
environment:
sdk: ">=3.0.0-218.1.beta <4.0.0"
However, my dummy code still wouldn't compile:
(double x, double y) geoLocation(String name) {
return (1, 3);
}
VSC would show me this error message:
This requires the 'records' language feature to be enabled.
Try updating your pubspec.yaml to set the minimum SDK constraint to 3.0.0 or higher, and running 'pub get'.
I did already upgrade the minimum SDK constraint t0 3.0.0. So I looked for how to enable a language feature. It turns out you can do it in analysis_options.yaml.
analyzer:
enable-experiment:
- records
And now it works!
Running unit tests
Even though the Analyzer is correctly set up and VSC won't complain anymore, if I run the tests by running flutter test
, I still get this error:
$ flutter test
...
Error: This requires the experimental 'records' language feature to be enabled.
Try passing the '--enable-experiment=records' command line option.
In order to run unit tests with the command line, I should run flutter test --enable-experiment=records
instead.
Debugging the app
Finally when I try to run the App by typing F5, I get this error:
Launching lib/main.dart on sdk gphone64 arm64 in debug mode...
main.dart:1
: Error: This requires the experimental 'class-modifiers' language feature to be enabled.
transporter.dart:1
Try passing the '--enable-experiment=class-modifiers' command line option.
abstract interface class Transporter {
^^^^^^^^^
Target kernel_snapshot failed: Exception
2
FAILURE: Build failed with an exception.
* Where:
Script '/Users/anhtuan/flutter/packages/flutter_tools/gradle/flutter.gradle' line: 1154
* What went wrong:
Execution failed for task ':app:compileFlutterBuildDebug'.
I tried to look at flutter.gradle but there is nothing very informative in there.
So I tried adding a new configuration:
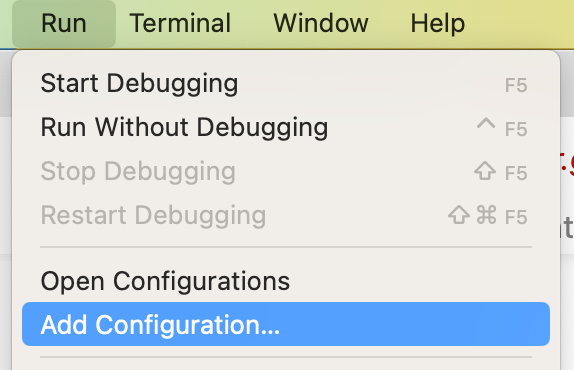
It created the file .vscode/launch.json with the following content:
{
// Use IntelliSense to learn about possible attributes.
// Hover to view descriptions of existing attributes.
// For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387
"version": "0.2.0",
"configurations": [
{
"name": "cargo",
"request": "launch",
"type": "dart"
},
{
"name": "cargo (profile mode)",
"request": "launch",
"type": "dart",
"flutterMode": "profile"
},
{
"name": "cargo (release mode)",
"request": "launch",
"type": "dart",
"flutterMode": "release"
}
]
}
I tried adding a key, and it autocompleted it for me:
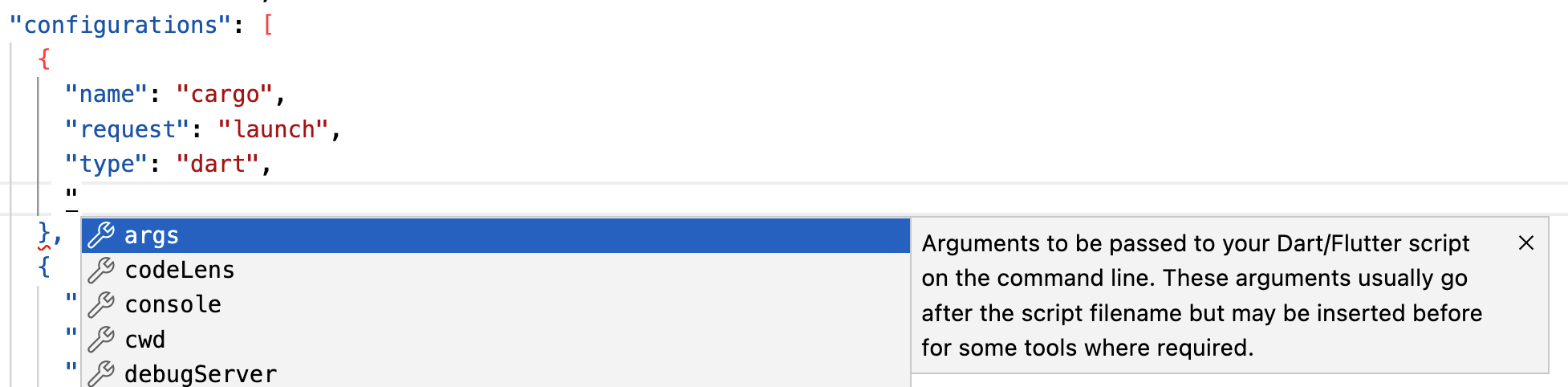
So I added this: "args": ["--enable-experiment=class-modifiers"]
and ran it again in Debug Mode and... it worked!